Virtual Card Creation
With the Create Virtual Card API, you can create virtual cards that your customers can use instantly once they have been created/issued and funded.
Create a card holder
To successfully create a virtual card, you must first create a card holder, the user to whom the card will be issued. A card holder can request and manage more than one virtual card.
To create a card holder, make a POST request to the endpoint:
{{baseurl}}/api/v1/cardholders
These are the parameters of the request body:
Field | Data Type | Description |
---|---|---|
first_name | String | Required. The first name on the card |
last_name | String | Required. The last name on the card |
email | String | Required. The email of the card holder |
phone | String | Optional. The card holder's phone number |
date_of_birth | String | Required. The date of birth of the card holder |
address | Object | Required. |
address.street | String | Required. The street address of the card holder |
address.city | String | Required. The city address of the card holder |
address.state | String | Required. The state address of the card holder |
address.country | String | Required. The country address of the card holder |
address.zip_code | String | Required. The zip code of the card holder's address |
country_identity | Object | Required. |
country_identity.type | String | Required. This specifies the type of identification that applies to the card holder's country. The available type is bvn |
country_identity.number | String | Required. This specifies the unique identity number for the card holder's country_identity.type . |
identity | Object | Required. |
identity.type | String | Required. The type of identity document submitted by the card holder. Options include nin , voters_card , drivers_license , passport . |
identity.number | String | Required. The identity document number of the card holder. |
identity.image | String - Base64 | Required. The identity document image |
identity.country | String | Required. (ISO3166 alpha-2, e.g NG for Nigeria) The country as specified on the identity document. |
This is what a typical response would look like:
{
"first_name": "Onyx",
"last_name": "Sanders",
"email": "[email protected]",
"phone_number": "+2348030537445",
"date_of_birth": "2002-11-11",
"country_identity": {
"type": "bvn",
"number": "22345678356"
},
"address": {
"street": "No 14, Kora street",
"city": "Lekki",
"state": "Lagos",
"country": "NG",
"zip_code": "101234"
},
"identity": {
"type": "nin",
"number": "53748374688",
"image": "/9j/4AAQSkZJRgABAQEASABIAAD/4gHYSUNDX1BST0ZJTEUAAQEAAAHIAAAAAAQwAABtb",
"country": "NG"
}
}
Create a virtual card (via API)
Once you have created a card holder, you will be able to issue virtual cards for that card holder. These virtual cards must be associated with a Visa or MasterCard scheme. You can choose the card scheme you want for a virtual card upon creation. However, if no scheme is selected, the MasterCard scheme will be assigned to the card by default.
Always ensure that you have sufficient funds in your issuing balance for card creation.
Use this request to create cards:
{{baseurl}}/api/v1/cards
The request body should have the following parameters:
Field | Data Type | Description |
---|---|---|
currency | String | Required. This is the currency under which the card is created. (USD ) |
card_holder_reference | String | Required. This is the unique reference of the cardholder. |
reference | String | Required. This is your unique reference for the card. |
type | String | Required. This is the type of card to be created. Can be physical or virtual . However, only virtual cards can be issued for now. |
brand | String | Required. This is the card brand or scheme - mastercard or visa . |
With a successful request, this is an example of what the card creation response would look like:
{
"status": true,
"message": "Card creation pending",
"data": {
"reference": "226eeb6f-f6cb-562f-a5e8-4uhsdechhgtywhg",
"currency": "USD",
"type": "virtual",
"holder_name": "Onyx Sanders",
"status": "pending"
}
}
A webhook notification will also be sent to your application immediately the virtual card is created. The webhook notification request will look like this:
{
"event": "card.creation.success",
"data": {
"reference": "webhook unique reference",
"card_reference": "876eeb6f-f6cb-562f-a5e8-48d91dec7999",
"type": "virtual",
"currency": "USD",
"holder_name": "John Doe",
"brand": "visa",
"expiry_month": "09",
"expiry_year": "25",
"first_six": "111111",
"last_four": "6777",
"status": "active",
"date": "2000-11-11T10:00:00"
}
}
Virtual card details
Once you have successfully created a virtual card, you can retrieve the details of the card.
The request to get the details of a virtual card is:
{{baseurl}}/api/v1/cards/:reference
And the response would look like this:
{
"status": true,
"message": "Card details retrieved successfully",
"data": {
"reference": "Card-Ref-0000-0011",
"type": "virtual",
"first_six": "111111",
"last_four": "6714",
"pan": "1111115476876714",
"cvv": "123",
"brand": "visa",
"expiry_month": "04",
"expiry_year": "2023",
"currency": "USD",
"balance": 0,
"status": "active",
"billing": {
"city": "Dr Doe City",
"state": "LA",
"country": "NG",
"address1": "No 78, doe street",
"address2": "doe boulevard",
"zip_code": "102408"
},
"card_holder": {
"first_name": "John",
"last_name": "Doe",
"email": "[email protected]",
"phone_number": "2348012345678",
"address": {
"city": "Lekki",
"state": "Lagos",
"street": "No 14, Doe street",
"country": "NG",
"zip_code": "101234"
}
},
"date_created": "2023-04-06T15:33:54.000Z"
}
}
Fetching all cards
You can retrieve all the virtual cards you have created by making a GET request to the following endpoint via API:
{{baseurl}}/api/v1/cards
The API also accepts request parameters. For instance, {{baseurl}}/api/v1/cards?status=active
retrieves all active cards that you have created.
Parameter | Type | Required? | Description |
---|---|---|---|
status | String | false | This is the status of the card. It can be any one of active , terminated , pending , deactivated , or expired . |
type | String | false | This is the type of cards - virtual or physical . At the moment, only virtual cards are available. |
start_date | String | false | This is the specific date from which you would like the query to start. The expected date format is YYYY-MM-DD. |
end_date | String | false | This is the specific end date for the search query. The expected date format is YYYY-MM-DD. |
See below an example of a response for this request:
{
"status": true,
"message": "Cards retrieved successfully",
"data": {
"data": [
{
"reference": "cd-rf—John-doe",
"type": "virtual",
"currency": "USD",
"first_six": "111111",
"last_four": "6714",
"status": "active",
"holder_name": "John Doe",
"brand": "visa",
"expiry_month": "04",
"expiry_year": "2023",
"date_created": "2023-04-06T15:33:54.000Z"
},
],
"paging": {
"total_items": 1,
"page_size": 10,
"current": 1,
"count": 1
}
}
}
You can view and manage all the virtual cards you have created from your Kora dashboard. Additionally, you can see the details of a specific card.
To view all the cards you have created, go to the Issuing page and navigate to the Issued Cards tab. Clicking on a card will display its details.
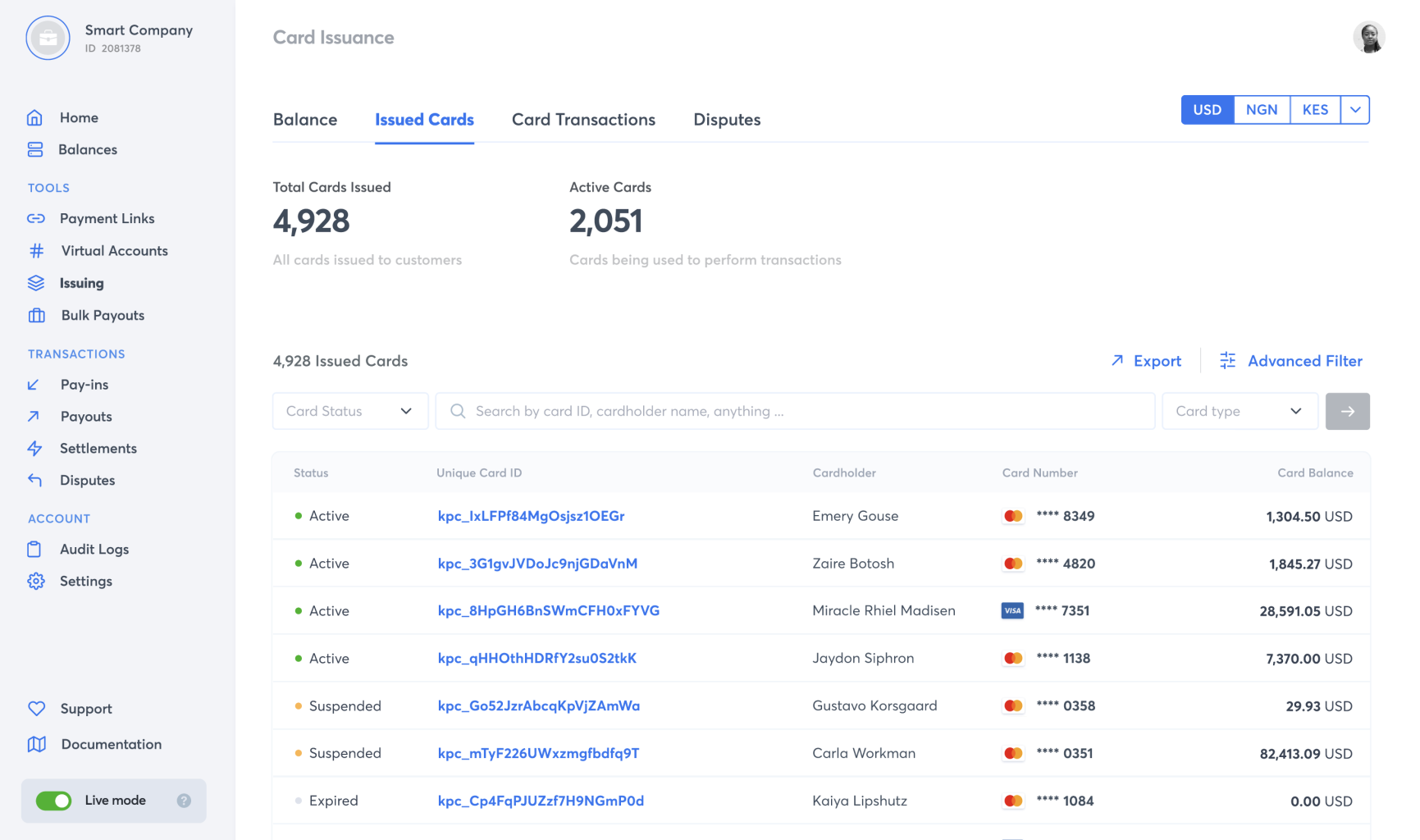
On the card details page, you can view the card transactions, balance, and events.
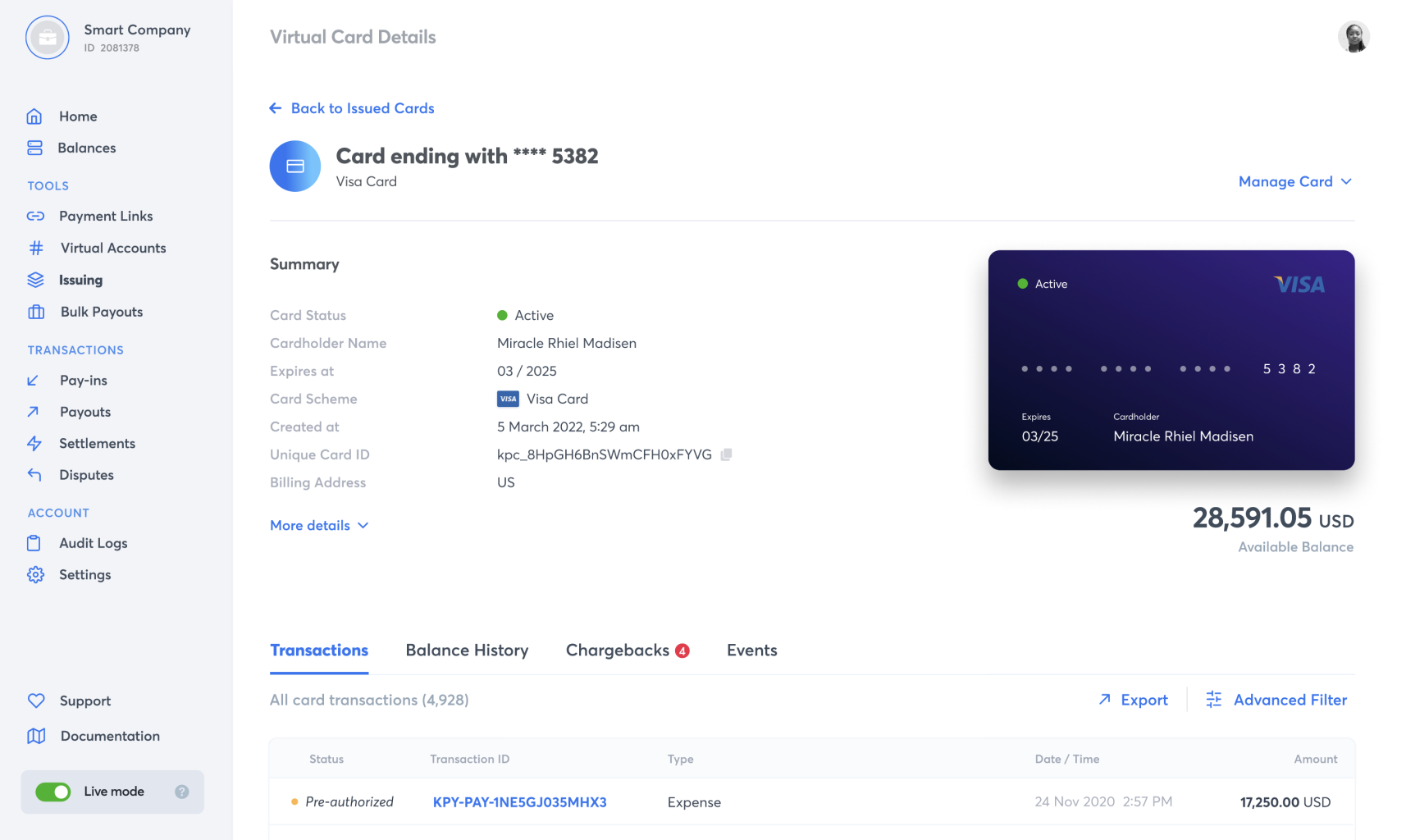
Updated 9 months ago